Learn C Programming Language
The Queue in C programming
Queues
A queue is similar to a checkout line in a grocery store—the first person in line is serviced first, and other customers enter the line only at the end and wait to be serviced.
Queue nodes are removed only from the head of the queue and are inserted only at the tail(rear) of the queue . For this reason, a queue is referred to as a first-in, first-out (FIFO) data structure.
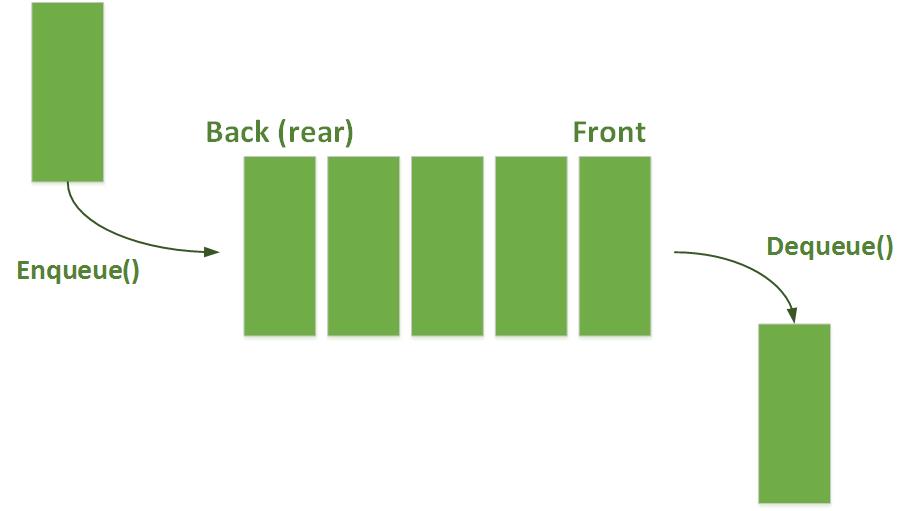
The insert and remove operations are known as enqueue and dequeue, respectively.
Function enqueue
Function enqueue receives three arguments: the address of the pointer to the head of the queue, the address of the pointer to the tail of the queue and the value to be inserted in the queue.
// insert a node in at queue tail
void enqueue( QueueNodePtr *headPtr, QueueNodePtr *tailPtr,
char value ){
QueueNodePtr newPtr; // pointer to new node
newPtr = malloc( sizeof( QueueNode ) );
if ( newPtr != NULL ) { // is space available
newPtr->data = value;
newPtr->nextPtr = NULL;
// if empty, insert node at head
if ( isEmpty( *headPtr ) ) {
*headPtr = newPtr;
} // end if
else {
( *tailPtr )->nextPtr = newPtr;
} // end else
*tailPtr = newPtr;
} // end if
else {
printf( "%c not inserted. No memory available.\n", value );
} // end else
} // end function enqueue
Function dequeue
Function dequeue receives the address of the pointer to the head of the queue and the address of the pointer to the tail of the queue as arguments and removes the first node from the queue.
// remove node from queue head
char dequeue( QueueNodePtr *headPtr, QueueNodePtr *tailPtr ){
char value; // node value
QueueNodePtr tempPtr; // temporary node pointer
value = ( *headPtr )->data;
tempPtr = *headPtr;
*headPtr = ( *headPtr )->nextPtr;
// if queue is empty
if ( *headPtr == NULL ) {
*tailPtr = NULL;
} // end if
free( tempPtr );
return value;
} // end function dequeue
Note: to run the program you need to build first a main menu using the switch (choice) statement.
Ads Right