Learn C Programming Language
Random-Access Files in C programming
Random-Access File
Individual records of a random-access file are normally fixed in length and may be accessed directly (and thus quickly) without searching through other records.
This makes random-access files appropriate for :
- airline reservation systems
- banking systems
- point-of-sale systems
- other kinds of transaction-processing systems that require rapid access to specific data.
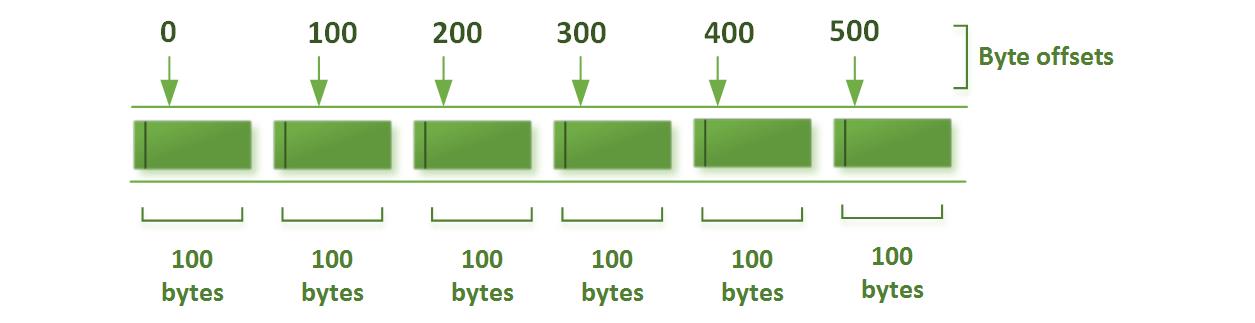
Fixed-length records enable data to be inserted in a random-access file without destroying other data in the file. Data stored previously can also be updated or deleted without rewriting the entire file.
Creating a Random-Access File
Functions fwrite and fread are capable of reading and writing arrays of data to and from disk. The third argument of both fread and fwrite is the number of elements in the array that should be read from or written to disk.
The following program open a random-access file, define a record format using a struct, write data to the disk and close the file.
// Creating a random-access file sequentially
#include <stdio.h>
// clientData structure definition
struct clientData {
unsigned int acctNum; // account number
char lastName[ 15 ]; // account last name
char firstName[ 10 ]; // account first name
double balance; // account balance
}; // end structure clientData
int main( void ) {
unsigned int i; // counter used to count from 1-100
// create clientData with default information
struct clientData blankClient = { 0, "", "", 0.0 };
FILE *cfPtr; // credit.dat file pointer
// fopen opens the file; exits if file cannot be opened
if ( ( cfPtr = fopen( "credit.dat", "wb" ) ) == NULL ) {
puts( "File could not be opened." );
} // end if
else {
// output 100 blank records to file
for ( i = 1; i <= 100; ++i ) {
fwrite( &blankClient, sizeof( struct clientData ), 1, cfPtr );
} // end for
fclose ( cfPtr ); // fclose closes the file
} // end else
} // end main
Writing Data Randomly to a Random-Access File
The program writes data to the file "credit.dat". It uses the combination of fseek and fwrite to store data at specific locations in the file.
Function fseek sets the file position pointer to a specific position in the file, then fwrite writes the data.
// Writing data randomly to a random-access file
#include <stdio.h>
// clientData structure definition
struct clientData {
unsigned int acctNum; // account number
char lastName[ 15 ]; // account last name
char firstName[ 10 ]; // account first name
double balance; // account balance
}; // end structure clientData
int main( void ) {
FILE *cfPtr; // credit.dat file pointer
// create clientData with default information
struct clientData client = { 0, "", "", 0.0 };
// fopen opens the file; exits if file cannot be opened
if ( ( cfPtr = fopen( "credit.dat", "rb+" ) ) == NULL ) {
puts( "File could not be opened." );
} // end if
else {
// require user to specify account number
printf( "%s", "Enter account number"
" ( 1 to 100, 0 to end input )\n? " );
scanf( "%d", &client.acctNum );
// user enters information, which is copied into file
while ( client.acctNum != 0 ) {
// user enters last name, first name and balance
printf( "%s", "Enter lastname, firstname, balance\n? " );
// set record lastName, firstName and balance value
fscanf( stdin, "%14s%9s%lf", client.lastName,
client.firstName, &client.balance );
// seek position in file to user-specified record
fseek( cfPtr, ( client.acctNum - 1 ) *
sizeof( struct clientData ), SEEK_SET );
// write user-specified information in file
fwrite( &client, sizeof( struct clientData ), 1, cfPtr );
// enable user to input another account number
printf( "%s", "Enter account number\n? " );
scanf( "%d", &client.acctNum );
} // end while
fclose( cfPtr ); // fclose closes the file
} // end else
} // end main
Output:
Enter account number ( 1 to 100, 0 to end input )
? 37
Enter lastname, firstname, balance
? Barker Doug 0.00
Enter account number
? 29
Enter lastname, firstname, balance
? Brown Nancy -24.54
Enter account number
? 96
Enter account number
? 0
Ads Right